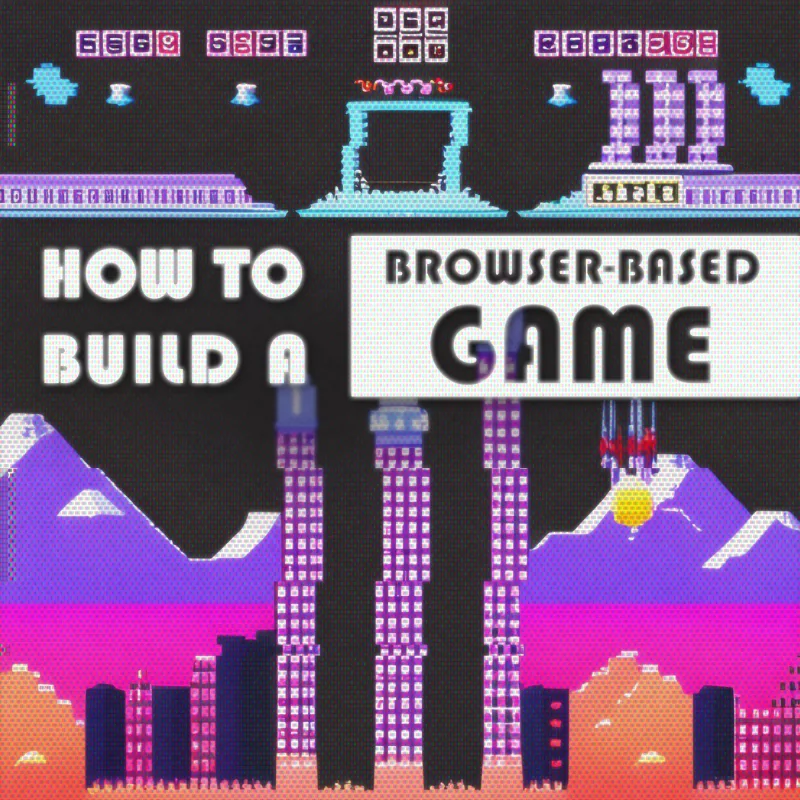
Browser-based games have gained immense popularity, captivating players of all ages and providing hours of entertainment. These games, accessible directly through web browsers, offer a convenient and engaging experience without the need for additional software installations. One of the key technologies behind the creation of these games is JavaScript, a versatile programming language that powers interactive and dynamic web content. With JavaScript, developers can craft captivating and immersive gaming experiences right within the browser.
Building browser-based games with JavaScript opens up a world of possibilities. It allows developers to leverage the ubiquity of web browsers and reach a wide audience across different devices and platforms. From simple puzzle games to complex multiplayer adventures, the flexibility of JavaScript empowers developers to bring their creative visions to life.
This guide will walk you through the process of building a working browser-based game with JavaScript, from starting with the fundamentals, to implementing game logic. You will even find helpful code examples and a complete working game demo with source code. Whether you’re a seasoned developer looking to venture into the world of browser-based game development or a beginner with a passion for coding, this article will provide you with the essential knowledge and practical techniques to start creating a compelling browser-based game.
Advantages of Browser-based Games
Browser-based games, as the name suggests, are games that can be played directly within a web browser without the need for downloading or installing additional software. They leverage the power of web technologies, such as HTML, CSS, and JavaScript, to deliver interactive and engaging gaming experiences.
One of the key advantages of using JavaScript for browser-based game development is its widespread adoption and compatibility. JavaScript is supported by all major web browsers, making it accessible to a vast number of users across different devices and platforms. This means that your game can reach a wide audience without the need for users to install specific software or plugins.
Moreover, JavaScript offers a rich set of features and functionalities that are well-suited for game development. Its versatility allows developers to handle game logic, user input, animations, and more, all within the browser environment. With the advent of modern web technologies, JavaScript-powered games can achieve impressive visuals, smooth animations, and immersive gameplay.
The popularity of browser-based games continues to soar, thanks to their accessibility and the ease of sharing and playing games directly from a web browser. They provide instant entertainment without the barriers of installation or compatibility issues. Additionally, browser-based games can be seamlessly integrated into websites, making them an effective tool for engaging users and driving traffic to your online platforms.
Setting Up Your Development Environment
Before we dive into browser-based game development with JavaScript, it’s important to set up the right development environment. This will ensure a smooth and efficient workflow as you bring your game ideas to life.
Choose a Code Editor:
To write and manage your JavaScript code, you’ll need a reliable code editor. There are several options available, each with its own set of features and benefits. Popular choices among developers include Visual Studio, Sublime Text, and Atom. These editors provide syntax highlighting, code suggestions, and other helpful features that enhance your coding experience. Choose the code editor that suits your preferences and install it on your machine.
Configure JavaScript Libraries and Frameworks:
JavaScript libraries and frameworks can greatly simplify the development process and enhance the functionality of your game. Depending on the complexity of your game, you may choose to utilize libraries such as jQuery for DOM manipulation or Phaser for game-specific functionalities. Additionally, frameworks like React or Vue.js can be employed for building more complex game interfaces and managing state.
Designing the Game Concept
Now it’s time to get into the creative process of designing your game concept. This is where you define the genre, mechanics, and rules that will shape the gameplay experience. With careful planning and outlining, you can ensure an engaging and immersive game for your players.
Select a Game Genre and Concept:
Consider the type of game you want to create and the interests of your target audience. Are you envisioning a fast-paced action game, a strategic puzzle game, or perhaps an adventurous role-playing game? Think about what excites you as a developer and what will resonate with your players. Once you have a genre in mind, brainstorm unique and captivating concepts that will make your game stand out. Be creative and explore various ideas before settling on one that sparks your passion.
Outline Game Mechanics and Rules:
Game mechanics are the rules, actions, and interactions that define how your game works. Determine the core mechanics that will drive your gameplay, such as jumping, shooting, solving puzzles, or managing resources. Consider how these mechanics will challenge and engage players, ensuring a balance between difficulty and enjoyment. Outline the rules of your game, including win and lose conditions, scoring systems, and any special features or power-ups that will enhance the gameplay experience.
Creating the Game Structure
Now that you have a clear game concept in mind, it’s time to start building the foundation of your browser-based game. This is where we create the game structure, including setting up the game canvas and defining game states and screens.
Build the Game Canvas:
The game canvas is the area where all the game elements will be rendered and displayed. It acts as a visual container for your game graphics and provides the space where players will interact with your game. To create the game canvas, you can use HTML5’s <canvas>
element, which provides a drawing surface that you can manipulate using JavaScript. Set the canvas dimensions and position it appropriately within your webpage to ensure optimal visibility and responsiveness.
Set Up Game States and Screens:
Game states represent different stages or screens of your game, such as the main menu, level selection, gameplay, and game over screen. Each state has its own set of functionalities and visual elements. By structuring your game into states, you can easily manage the flow and transitions between different parts of the game. Determine the states your game will have and define the necessary functions and assets for each state. This will allow you to control the logic and visual representation of your game as players progress through different screens.
To create a basic game canvas using HTML5 and JavaScript, follow these steps:
- HTML Markup:
<canvas id="gameCanvas" width="400" height="400"></canvas>
- JavaScript Code:
const canvas = document.getElementById("gameCanvas");
const ctx = canvas.getContext("2d");
// Draw a background color
ctx.fillStyle = "black";
ctx.fillRect(0, 0, canvas.width, canvas.height);
In this example, we obtain the 2D rendering context (ctx) of the canvas, which allows us to draw on the canvas. We then use the fillRect method to draw a black background color on the entire canvas.
With the game canvas set up, you now have a visual space to bring your game to life.
Implementing Game Logic
With the game structure in place, it’s time to implement some game logic. This is where we set up user input and controls, code game interactions and actions.
Handling User Input and Controls:
User input and controls are crucial for player interaction and gameplay. You can capture user input, such as keyboard or mouse events, and use them to control the game elements. Map key presses or mouse movements to specific actions within your game, such as moving the player character or triggering game events.
Coding Game Interactions and Actions:
Game interactions and actions refer to the various behaviors and functionalities that occur during gameplay. This includes player movement, enemy AI, object interactions, scoring systems, and more. Use JavaScript to define the necessary functions and logic to control these interactions. Implement game rules, physics, animations, and any other game-specific mechanics to make your game dynamic and enjoyable.
To add player movement to your game, we can do something like this:
// Set up the initial player position
let playerX = canvas.width / 2;
let playerY = canvas.height / 2;
// Function to update the game state
function updateGame() {
// Clear the frame and color the canvas
context.fillStyle = "black";
context.fillRect(0, 0, canvas.width, canvas.height);
// Move the player based on arrow keys
const arrowKeySpeed = 5;
if (arrowKeys.left && playerX > 0) playerX -= arrowKeySpeed;
if (arrowKeys.up && playerY > 0) playerY -= arrowKeySpeed;
if (arrowKeys.right && playerX < canvas.width - 50) playerX += arrowKeySpeed;
if (arrowKeys.down && playerY < canvas.height - 50) playerY += arrowKeySpeed;
// Draw the player
context.fillStyle = "#fb6";
context.fillRect(playerX, playerY, 50, 50);
// Request the next animation frame
requestAnimationFrame(updateGame);
}
// Function to handle arrow key events
const arrowKeys = {
left: false,
up: false,
right: false,
down: false,
};
function handleKeyDown(event) {
event.preventDefault();
if (event.key === "ArrowLeft") arrowKeys.left = true;
if (event.key === "ArrowUp") arrowKeys.up = true;
if (event.key === "ArrowRight") arrowKeys.right = true;
if (event.key === "ArrowDown") arrowKeys.down = true;
}
function handleKeyUp(event) {
event.preventDefault();
if (event.key === "ArrowLeft") arrowKeys.left = false;
if (event.key === "ArrowUp") arrowKeys.up = false;
if (event.key === "ArrowRight") arrowKeys.right = false;
if (event.key === "ArrowDown") arrowKeys.down = false;
}
// Add event listeners for arrow key controls
document.addEventListener("keydown", handleKeyDown);
document.addEventListener("keyup", handleKeyUp);
// Start the game loop
updateGame();
Here we have defined a player object with properties for position, speed, and dimensions. We created a function that checks for specific keyboard input and tracks the state of pressed keys. Depending on the key pressed, the player’s position is updated accordingly. You can modify the movement speed and controls based on your game requirements.
Adding Visuals and Audio
Now we will explore how to enhance your browser-based game by adding visual and audio elements. Integrating graphics, animations, sound effects, and music can help create an immersive and captivating gaming experience for your players.
Integrating Graphics and Animations:
Graphics and animations play a vital role in bringing your game to life. You can use various techniques to incorporate visuals into your game, such as sprite sheets, image rendering, and canvas drawing. Sprite sheets allow you to efficiently manage and animate multiple game elements, including characters, objects, and backgrounds. Utilize JavaScript and CSS to control sprite animation sequences, position them within the game canvas, and handle rendering updates. Experiment with different visual styles and effects to create a visually appealing game environment.
Incorporating Sound Effects and Music:
Sound adds depth and atmosphere to your game. It can evoke emotions, enhance gameplay moments, and provide audio cues for important events. HTML5 offers built-in audio capabilities that enable you to play sound effects and background music. With JavaScript, you can control when and how these audio elements are triggered. Experiment with different sound effects to match specific game actions and transitions. Consider adding background music that complements the game’s theme and setting, immersing players further into the gaming experience.
Let’s take a look at a code example that demonstrates how to display sprites and play sound effects using JavaScript:
// Load sprite image
const spriteImage = new Image();
spriteImage.src = "path/to/sprite.png";
// Draw sprite on the canvas
function drawSprite(sprite, x, y) {
context.drawImage(
spriteImage,
sprite.x,
sprite.y,
sprite.width,
sprite.height,
x,
y,
sprite.width,
sprite.height
);
}
// Play sound effect
function playSoundEffect(jump_sound) {
const audio = new Audio("path/to/sound.wav");
audio.play();
}
// Usage
const playerSprite = {
x: 0,
y: 0,
width: 32,
height: 32,
};
// Inside the game loop
drawSprite(playerSprite, player.x, player.y);
playSoundEffect("jump_sound");
In this example, we load a sprite image and define a drawSprite function that draws the sprite on the game canvas at the specified position. You can customize the drawSprite function to handle different animation frames and sprite sequences. Additionally, we implement a playSoundEffect function that plays a sound effect when called. You can associate different sound effects with specific game events or actions.
Testing and Debugging
Once you have implemented the core features of your browser-based game, it’s crucial to thoroughly test and debug it to ensure a smooth and enjoyable gaming experience for your players.
Strategies for Testing Game Functionality
- Functional Testing: Conduct comprehensive testing of different game scenarios, interactions, and user inputs. Verify that the game mechanics and logic work as intended. Test various game states, transitions, and edge cases to uncover any potential bugs or glitches.
- Cross-Browser Testing: Test your game on multiple web browsers and versions to ensure compatibility and consistent behavior. Different browsers may interpret JavaScript and HTML5 features differently, so it’s essential to verify that your game functions correctly across a range of popular browsers.
- Device Testing: Test your game on different devices, including desktops, laptops, tablets, and mobile phones. Ensure that the game’s responsiveness, controls, and visuals adapt well to various screen sizes and orientations.
- User Testing: Gather feedback from a group of users or beta testers who can play your game and provide valuable insights. Observe their interactions, note any usability issues, and consider their suggestions for improvements.
Utilizing Browser Development Tools for Debugging
Modern web browsers offer powerful developer tools that can assist you in debugging your game. These tools provide features like console logging, breakpoints, network monitoring, and performance profiling. Here are a few commonly used browser development tools for debugging JavaScript games:
- Chrome DevTools: The DevTools console allows you to log messages, inspect variables, and track runtime errors. You can set breakpoints to pause the execution of your game and examine the state of variables and the call stack. The network panel helps you monitor resource loading and performance.
- Firefox Developer Tools: Similar to Chrome DevTools, Firefox Developer Tools offer debugging capabilities, including console logging, breakpoints, and network monitoring. The Performance panel provides insights into your game’s performance, highlighting potential bottlenecks.
- Safari Web Inspector: Safari’s Web Inspector features console logging, JavaScript debugging, and network monitoring. It also includes a timeline tool for performance analysis and resource utilization.
- Microsoft Edge DevTools: Microsoft Edge DevTools offer similar debugging features, including console logging, breakpoints, and network monitoring. The Performance tool allows you to profile your game’s performance and identify areas for optimization.
Optimizing Performance
To provide players with a seamless and enjoyable gaming experience, it’s important to optimize the performance of your browser-based game.
Techniques for Optimizing Game Speed and Responsiveness
- Efficient Rendering: Optimize the rendering process by reducing unnecessary redraws and minimizing the number of DOM manipulations. Use techniques like requestAnimationFrame to synchronize animations with the browser’s rendering loop, ensuring smooth visuals.
- Code Optimization: Review and optimize your game’s JavaScript code to eliminate bottlenecks and improve execution speed. Avoid redundant calculations, minimize the use of expensive operations, and consider using algorithms or data structures that offer better performance for specific tasks.
- Caching and Preloading: Implement caching techniques to store and reuse frequently accessed game assets, such as images, sounds, and scripts. Preload essential assets during the game’s initialization phase to reduce loading times during gameplay.
- Minification and Compression: Minify your JavaScript, CSS, and HTML files by removing unnecessary spaces, comments, and line breaks. Compress your assets, such as images and audio files, to reduce their file size without significant quality loss. Smaller file sizes result in faster loading times for your game.
- Asynchronous Loading: Load game assets asynchronously to prevent blocking the main thread and improve perceived performance. Use techniques like lazy loading or dynamic loading to fetch assets on-demand, reducing the initial loading time.
Managing Memory Usage and Resource Consumption
- Resource Pooling: Implement resource pooling techniques to reuse and manage resources efficiently. Objects like bullets, enemies, or particles can be pooled and recycled instead of creating and destroying them repeatedly, reducing memory allocation and garbage collection overhead.
- Memory Management: Be mindful of memory usage throughout your game. Dispose of unused objects, remove event listeners, and release references to allow the garbage collector to reclaim memory. Avoid memory leaks by properly managing object lifecycles.
- Asset Optimization: Optimize your game’s assets, such as images and sounds, to reduce their memory footprint. Use appropriate image formats and compression techniques to balance file size and visual quality. Consider using audio formats with efficient compression to minimize memory usage.
Finish Your Game
Using these techniques and some more JavaScript magic, we’ve built a simple working browser-based game prototype! We have a player object (orange square) with the objective of hitting a target (grey square). Feel free to use the arrow keys to play around with it:
And if you’re interested, here is the full source code:
// Get the canvas element
const canvas = document.getElementById("gameCanvas");
const context = canvas.getContext("2d");
// Set up the initial player position
let playerX = canvas.width / 2 - 25;
let playerY = canvas.height / 2 - 25;
// Set up the initial target position
let targetX = getRandomPosition(canvas.width - 30);
let targetY = getRandomPosition(canvas.height - 30);
// Keep track of the touch position
let touchX = null;
let touchY = null;
// Function to update the game state
function updateGame() {
// Clear the frame and color the canvas
context.fillStyle = "black";
context.fillRect(0, 0, canvas.width, canvas.height);
// Move the player based on arrow keys
const arrowKeySpeed = 5;
if (arrowKeys.left && playerX > 0) playerX -= arrowKeySpeed;
if (arrowKeys.up && playerY > 0) playerY -= arrowKeySpeed;
if (arrowKeys.right && playerX < canvas.width - 50) playerX += arrowKeySpeed;
if (arrowKeys.down && playerY < canvas.height - 50) playerY += arrowKeySpeed;
// Move the player based on touch position
if (touchX !== null && touchY !== null) {
const touchSpeed = 5;
if (touchY < playerY) playerY -= touchSpeed;
if (touchY > playerY) playerY += touchSpeed;
if (touchX < playerX) playerX -= touchSpeed;
if (touchX > playerX) playerX += touchSpeed;
}
// Draw the player
context.fillStyle = "#fb6";
context.fillRect(playerX, playerY, 50, 50);
// Draw the target
context.fillStyle = "#ccc";
context.fillRect(targetX, targetY, 30, 30);
// Check for collision between player and target
if (checkCollision(playerX, playerY, 50, 50, targetX, targetY, 30, 30)) {
// Generate new target position
targetX = getRandomPosition(canvas.width - 30);
targetY = getRandomPosition(canvas.height - 30);
// Play sound effect
playSoundEffect("pop");
}
// Request the next animation frame
requestAnimationFrame(updateGame);
}
// Function to handle arrow key events
const arrowKeys = {
left: false,
up: false,
right: false,
down: false,
};
function handleKeyDown(event) {
event.preventDefault();
if (event.key === "ArrowLeft") arrowKeys.left = true;
if (event.key === "ArrowUp") arrowKeys.up = true;
if (event.key === "ArrowRight") arrowKeys.right = true;
if (event.key === "ArrowDown") arrowKeys.down = true;
}
function handleKeyUp(event) {
event.preventDefault();
if (event.key === "ArrowLeft") arrowKeys.left = false;
if (event.key === "ArrowUp") arrowKeys.up = false;
if (event.key === "ArrowRight") arrowKeys.right = false;
if (event.key === "ArrowDown") arrowKeys.down = false;
}
// Function to handle touch events
function handleTouch(event) {
event.preventDefault();
const rect = canvas.getBoundingClientRect();
touchX = event.touches[0].clientX - rect.left;
touchY = event.touches[0].clientY - rect.top;
}
function handleTouchEnd() {
touchX = null;
touchY = null;
}
// Check for collision between two rectangles
function checkCollision(rect1X, rect1Y, rect1Width, rect1Height, rect2X, rect2Y, rect2Width, rect2Height) {
return (
rect1X < rect2X + rect2Width &&
rect1X + rect1Width > rect2X &&
rect1Y < rect2Y + rect2Height &&
rect1Y + rect1Height > rect2Y
);
}
// Generate a random position within a given range
function getRandomPosition(max) {
return Math.floor(Math.random() * max);
}
// Add event listeners for arrow key controls
document.addEventListener("keydown", handleKeyDown);
document.addEventListener("keyup", handleKeyUp);
// Add event listeners for touch controls
canvas.addEventListener("touchstart", handleTouch);
canvas.addEventListener("touchmove", handleTouch);
canvas.addEventListener("touchend", handleTouchEnd);
// Play sound effect
function playSoundEffect(pop) {
const audio = new Audio("https://photricity.com/wp-content/uploads/pop-sound-effect.mp3");
audio.play();
}
// Start the game loop
updateGame();