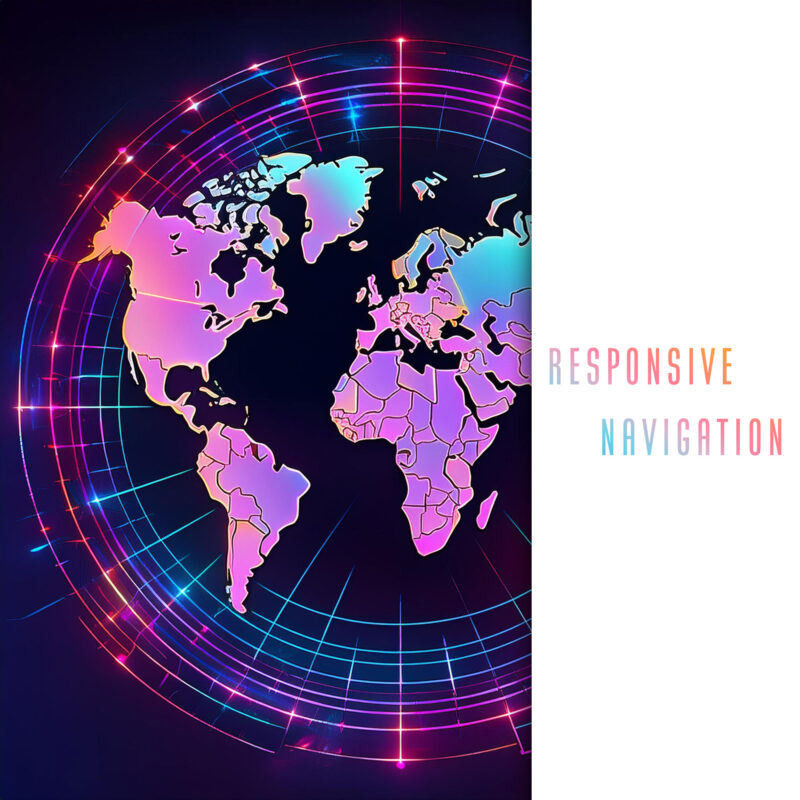
Imagine walking into a restaurant where the menu automatically adjusts based on whether you’re in the mood for a quick bite or a full-course meal. If you’re in a hurry, it highlights the appetizers and daily specials. If you’re ready to indulge, it elegantly expands to showcase the chef’s signature dishes. That’s what a responsive navigation menu does for your website—tailors the browsing experience to fit the user’s needs, whether they’re on a desktop, tablet, or smartphone. It’s about serving up the perfect menu of options for every digital diner who visits your site, ensuring that everyone leaves satisfied, no matter the device they use.
A responsive navigation menu is much more than a fancy gadget in your web design toolkit; it’s your user’s personal guide in the vast and often chaotic digital landscape. As screen sizes shrink and expand from desktop giants to palm-sized smartphones, your site’s navigation should effortlessly adjust to meet each device’s needs.
Why is this crucial? Because in today’s world, a frustrating navigation experience is like a bad handshake—it’s awkward and starts things off on the wrong foot. Ensuring your menu morphs gracefully across devices enhances user experience, boosts accessibility, and keeps visitors coming back. It’s not just about making things look pretty; it’s about creating a fluid, intuitive pathway that makes your visitors feel right at home, whether they’re clicking on a desktop or tapping on a smartphone. Let’s dive in and see how you can roll out the red carpet for every device visiting your site.
Understanding Responsive Design
Responsive design is like the Swiss Army knife of web development—it’s adaptable, versatile, and essential for any web project. At its core, responsive design is all about crafting websites that deliver an optimal viewing experience across a wide range of devices. Whether your visitor is on a 27-inch monitor or a 5-inch smartphone, your site should look and function seamlessly. Think of it as a chameleon that effortlessly blends into its surroundings, ensuring your content is always accessible and visually appealing, no matter the screen size.
Role of Navigation in Responsive Design
A well-crafted responsive navigation menu is crucial because it significantly impacts usability and accessibility. Imagine trying to find your way through a city with unclear signs—it’s frustrating and inefficient. The same goes for web navigation. A responsive menu ensures users can easily find what they’re looking for, whether they’re on a desktop, tablet, or smartphone.
But it’s not just about resizing elements. Responsive navigation must also adapt its behavior. On a desktop, you might have a horizontal menu with dropdowns. On a mobile device, that same menu might transform into a sleek hamburger icon that expands into a vertical list. This transformation ensures that navigation remains intuitive and user-friendly, enhancing the overall experience.
Moreover, accessibility is a key player in responsive design. A responsive navigation menu should be easy to navigate not only for users with different devices but also for those using assistive technologies. This means considering keyboard navigation, screen reader compatibility, and ensuring that all interactive elements are easily reachable and usable.
In a nutshell, responsive navigation is about more than just shrinking or expanding; it’s about rethinking how users interact with your site across different devices. It’s the difference between a smooth, enjoyable journey and a bumpy, confusing ride.
Less is More
Before you start crafting your navigation menu, let’s address the burning question: what links should you include? Imagine your website as a bustling city, and your navigation menu is the city’s map. You don’t want it cluttered with every alley and dead-end; instead, focus on the main roads that lead to important destinations.
Start by putting yourself in your users’ shoes (or screens). What are they here for? What paths do they need to take to find the most valuable content? Whether it’s the homepage, about page, services, or contact information, these should be your primary links. Keep it simple—think essential, not exhaustive. The goal is to make navigation intuitive, not overwhelming.
Basic HTML and CSS for Navigation Menus
Start with the basics: Create a nav
element to wrap your entire menu. This helps with semantics and accessibility.
Inside the nav
element, use an unordered list (<ul>
) to list out your navigation items. Each link goes inside a list item (<li>
) with an anchor tag (<a>
). A proper HTML structure helps search engines understand your site better.
Then, add classes to your elements to make styling them with CSS easier later on.
Here’s a simple example:
<nav class="navbar">
<ul class="nav-menu">
<li class="nav-item"><a href="#" class="nav-link">Home</a></li>
<li class="nav-item"><a href="#" class="nav-link">About</a></li>
<li class="nav-item"><a href="#" class="nav-link">Services</a></li>
<li class="nav-item"><a href="#" class="nav-link">Contact</a></li>
</ul>
</nav>
With the HTML structure in place, it’s time to bring our menu to life with some CSS. The goal here is to make the menu visually appealing and functionally robust.
- Resetting Styles: Start with a CSS reset to ensure consistency across different browsers.
- Basic Styling: Style the
nav
,ul
,li
, anda
elements to create a clean and simple navigation bar. - Flexbox Magic: Use Flexbox to align and distribute the menu items evenly. This makes the menu responsive and ensures it looks good on all screen sizes.
/* Reset some default styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
}
/* Base styles for larger screens */
.navbar {
background-color: #333;
padding: 1rem;
display: flex;
justify-content: space-between;
align-items: center;
}
.nav-menu {
display: flex;
list-style: none;
justify-content: space-around;
margin: 0;
padding: 0;
}
.nav-link {
color: white;
text-decoration: none;
font-size: 1.2rem;
padding: 0.5rem 1rem;
transition: background-color 0.3s ease;
}
.nav-link:hover {
background-color: #555;
border-radius: 5px;
}
In this CSS, we’re using Flexbox to create a horizontal layout for the menu items. The justify-content: space-around;
rule distributes the items evenly, ensuring a balanced look. The hover effect on the .nav-link
class gives a subtle but engaging feedback to the user, enhancing the overall experience.
Result:

Making Navigation Responsive
Let’s face it, a navigation menu that doesn’t adapt to different screen sizes is like a man trying to squeeze into his wife’s yoga pants—it’s just not a good fit. That’s where media queries come in, ensuring your navigation looks and works great on any device, from the smallest smartphone to the widest desktop monitor.
/* Media query for tablets and smaller screens */
@media (max-width: 768px) {
.nav-menu {
flex-direction: column;
align-items: center;
display: none; /* Hide menu by default */
width: 100%; /* Make it full width */
background-color: #333;
position: absolute;
top: 70px; /* Adjust position to align with navbar */
left: 0;
}
.nav-menu.active {
display: flex; /* Show menu when active */
}
.nav-link {
padding: 15px;
width: 100%;
text-align: center;
}
}
In this example, we’re using media queries to adjust the layout of the .nav-menu
and .nav-link
for different screen sizes. For smartphones and other small screens (max-width: 768px), the menu switches to a column layout.
JavaScript for Interactive Elements
CSS alone can’t handle all our responsive needs. To truly make our navigation menu user-friendly on smaller screens, we need a bit of JavaScript magic. Enter the hamburger menu—iconic, intuitive, and indispensable for mobile navigation.
JavaScript helps us toggle the visibility of the menu when the hamburger icon is clicked.
First, add the hamburger icon to your HTML:
<button class="hamburger" aria-label="Toggle navigation">
☰
</button>
A little CSS:
/* Hamburger button */
.hamburger {
background: none;
border: none;
color: white;
font-size: 2rem;
cursor: pointer;
display: none; /* Hidden on larger screens */
}
Next, add the following JavaScript to handle the menu toggle:
document.addEventListener('DOMContentLoaded', function () {
const hamburger = document.querySelector('.hamburger');
const navMenu = document.querySelector('.nav-menu');
hamburger.addEventListener('click', function () {
navMenu.classList.toggle('active');
});
});
This script listens for a click event on the hamburger button and toggles the active
class on the .nav-menu
element, showing or hiding the menu as needed. The active
class works in conjunction with the media queries we defined earlier to control the display of the menu.
Full example:
See the Pen Responsive Navigation Example by Josh Meyer (@Josh-Meyer) on CodePen.
With these responsive techniques, your navigation menu will be as flexible as a gymnast, gracefully adapting to any screen size. So go ahead, give it a try, and watch your menu transform into a user-friendly, device-agnostic masterpiece. And remember, in the world of responsive design, flexibility is key—both in code and in mindset.
Next-Level
When it comes to building responsive navigation menus, why reinvent the wheel? There are fantastic CSS frameworks and JavaScript libraries out there that can make your life a whole lot easier, and take your navigation to the next level. Think of them as your handy toolbox, filled with pre-built components and nifty features.
- Bootstrap: The granddaddy of CSS frameworks, Bootstrap is known for its robust, responsive grid system and pre-styled components. Its navigation bar (navbar) component is a breeze to implement and customize. With Bootstrap, you can get a fully functional and stylish navigation menu up and running in no time.
- Foundation: Another heavyweight in the CSS framework arena, Foundation offers flexible and customizable components for creating responsive menus. It’s especially known for its mobile-first approach and accessibility features.
- React: If you’re looking to build more dynamic and interactive navigation menus, React is your go-to JavaScript library. Its component-based architecture allows you to create reusable UI components, making your menu both modular and maintainable. Plus, there are tons of pre-built React components available for navigation menus.
Now that you’re equipped with all these tips and tools, it’s time to get creative. Don’t be afraid to experiment with different styles, layouts, and interactive features. The beauty of web development is that there’s always room for innovation. Customize these concepts to fit your project needs and make your navigation menu uniquely yours. Remember, the best way to learn is by doing, so dive in and have fun with it!